I was receiving interesting results while playing around with 2D perlin noise applied to shuffled gradient colours.
Will try to describe the process here.
Noise to Pixels
The code is drawing pixel-wise on a 2D array of thi.ng color and applies the finished image to the processing (quil) graphics at the end. To optimise for speed, which is definitely necessary for this case, i worked with plain arrays instead of the standard Clojure data structures. This needs quite some type hints in the Clojure code like:
(defn make-color-matrix [width height]
^"[[Lthi.ng.color.core.RGBA;" (make-array (Class/forName "thi.ng.color.core.RGBA") height width))
[[L stands for [][] in Java, the 2D array.
The full color matrix code can be found here.
The code to apply the 2D perlin noise is stolen from here.
Now we can apply a noise function
(defn noise-function [coloring-function octaves scale turb]
(let [r (rand 1000)]
(fn [^"[[Lthi.ng.color.core.RGBA;" mt x y]
(let [[x' y'] (map #(+ r %) (turb x y))
n (noise/octave-noise2 x' y' scale octaves)
newcolor (coloring-function n)]
(cm/aset2c mt x y newcolor)))))
to the color matrix.
Colouring the Noise
To color the image is first set up a palette
(def palette_1 [(c/as-rgba (c/css "rgba(51,92,103,1)"))
(c/as-rgba (c/css "rgba(255,243,176,1)"))
(c/as-rgba (c/css "rgba(224,159,62,1)"))
(c/as-rgba (c/css "rgba(158,42,43,1)"))
(c/as-rgba (c/css "rgba(84,11,14,1)"))])
and created a gradient of two random colours of the palette
(defn random-grad [palette steps]
(grad/cosine-gradient
steps
(grad/cosine-coefficients (rand-nth palette) (rand-nth palette))))
…shuffling the gradient colours…
(defn shuffled-random-grad [palette steps] (shuffle (random-grad palette steps)))
The final colouring function is taking n between -1 and 1 from the noise function and applies different shuffled gradients to different ranges of the noise:
(defn get-coloring-function []
(let [grad1 (shuffled-random-grad palette_1 40)
grad2 (shuffled-random-grad palette_1 40)
grad3 (shuffled-random-grad palette_1 40)]
(fn [n]
(cond
(< n -0.15) (pth grad1 n -1 -0.15)
(< n 0.15) (pth grad2 n -0.15 0.15)
(>= n 0.15) (pth grad3 n 0.15 1)))))
This already leads to interesting images.
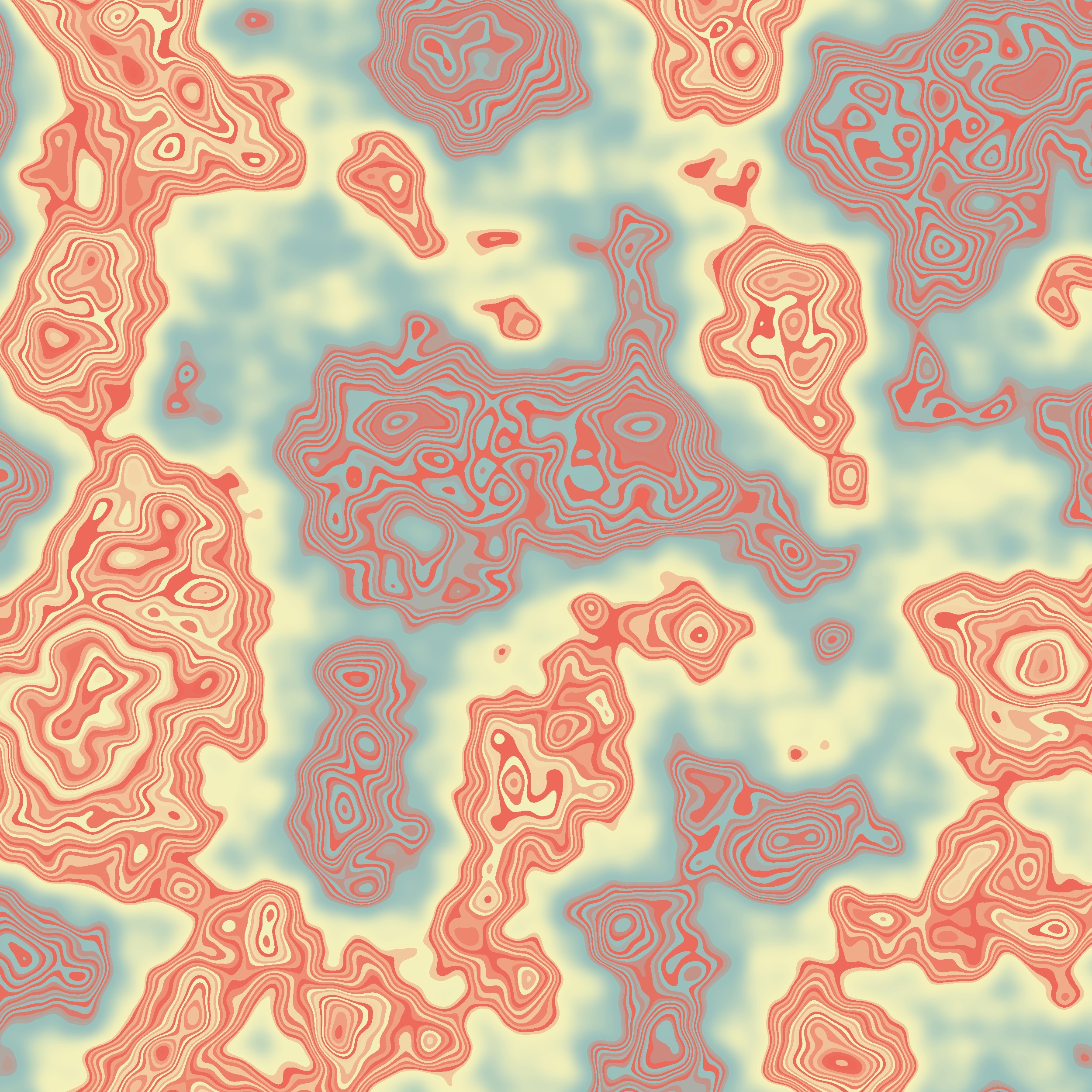
Finally I put some an offset to the y-axis which gives the image an additional element of vertical lines. Accidentally stumbled over this last element 😉
Final Images
The images below are all randomly generated without changing the code in between.
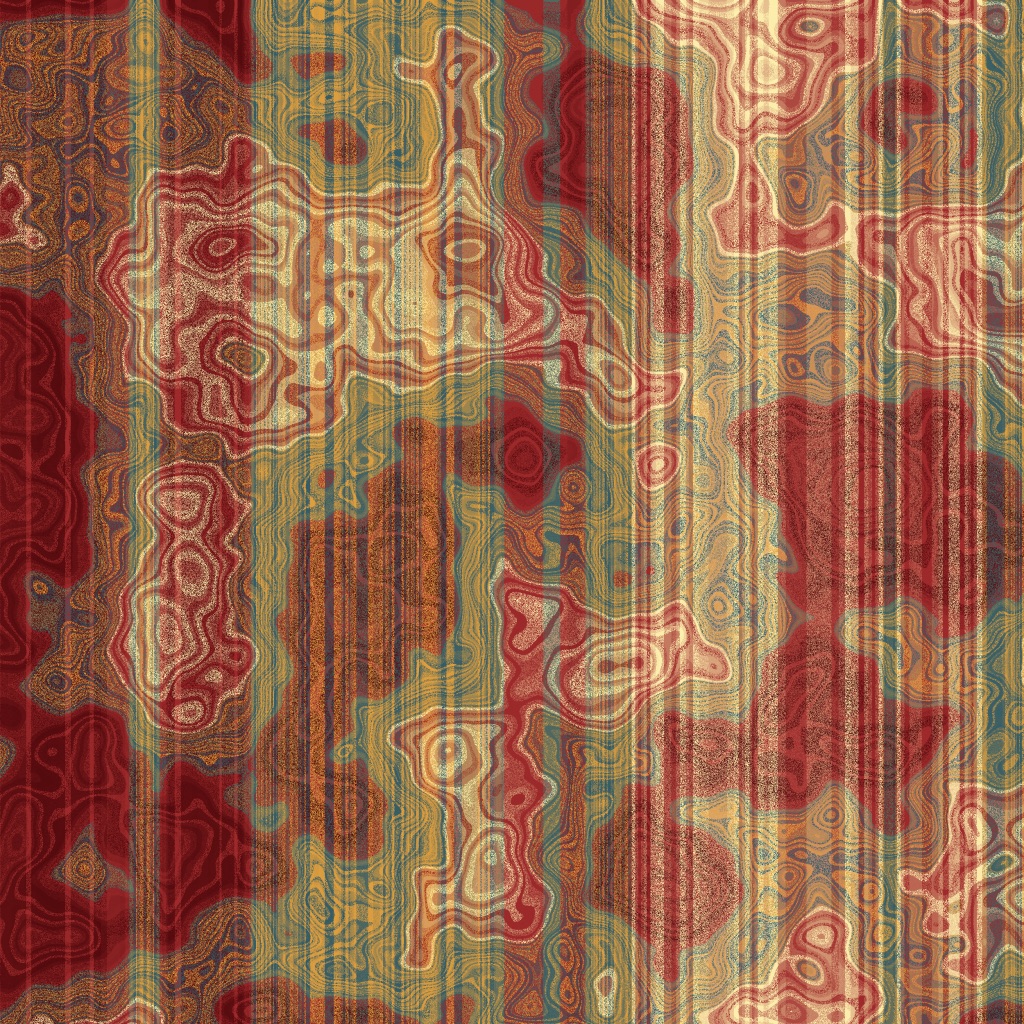
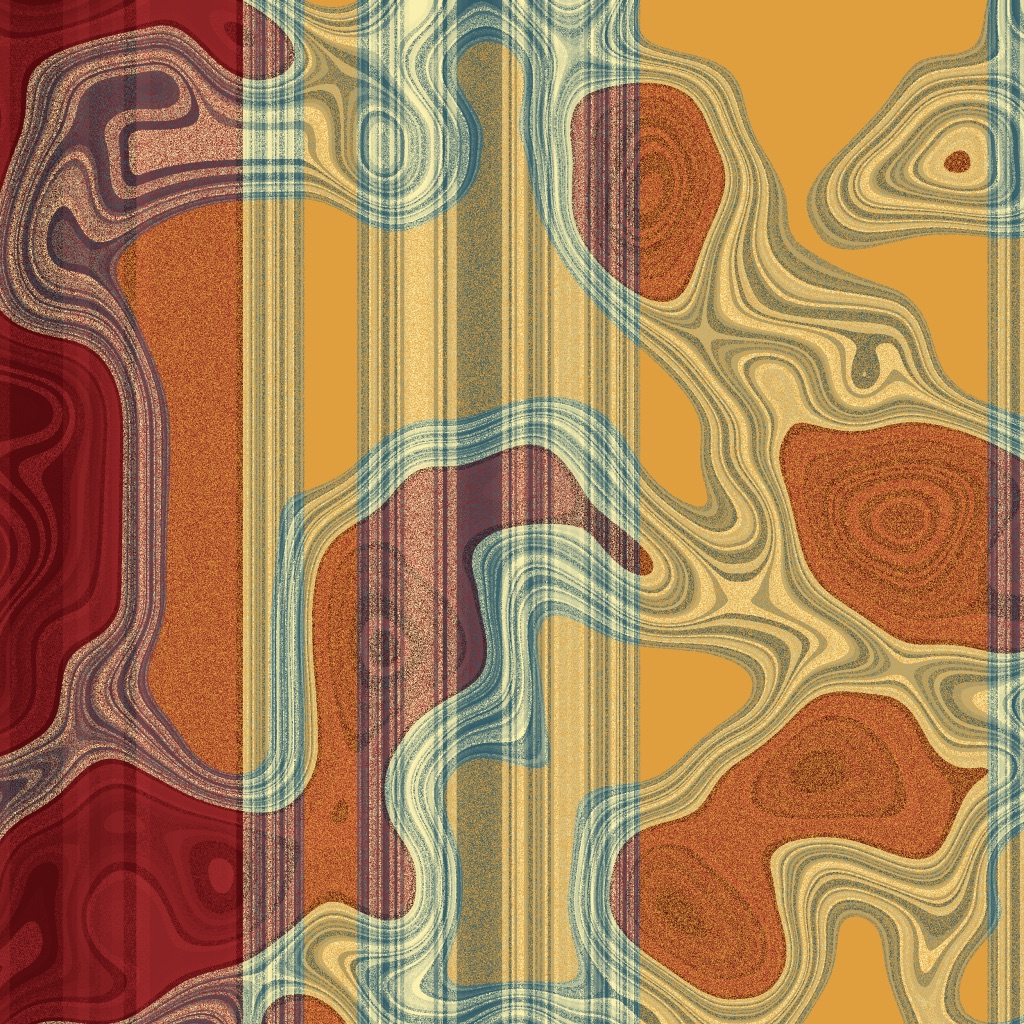
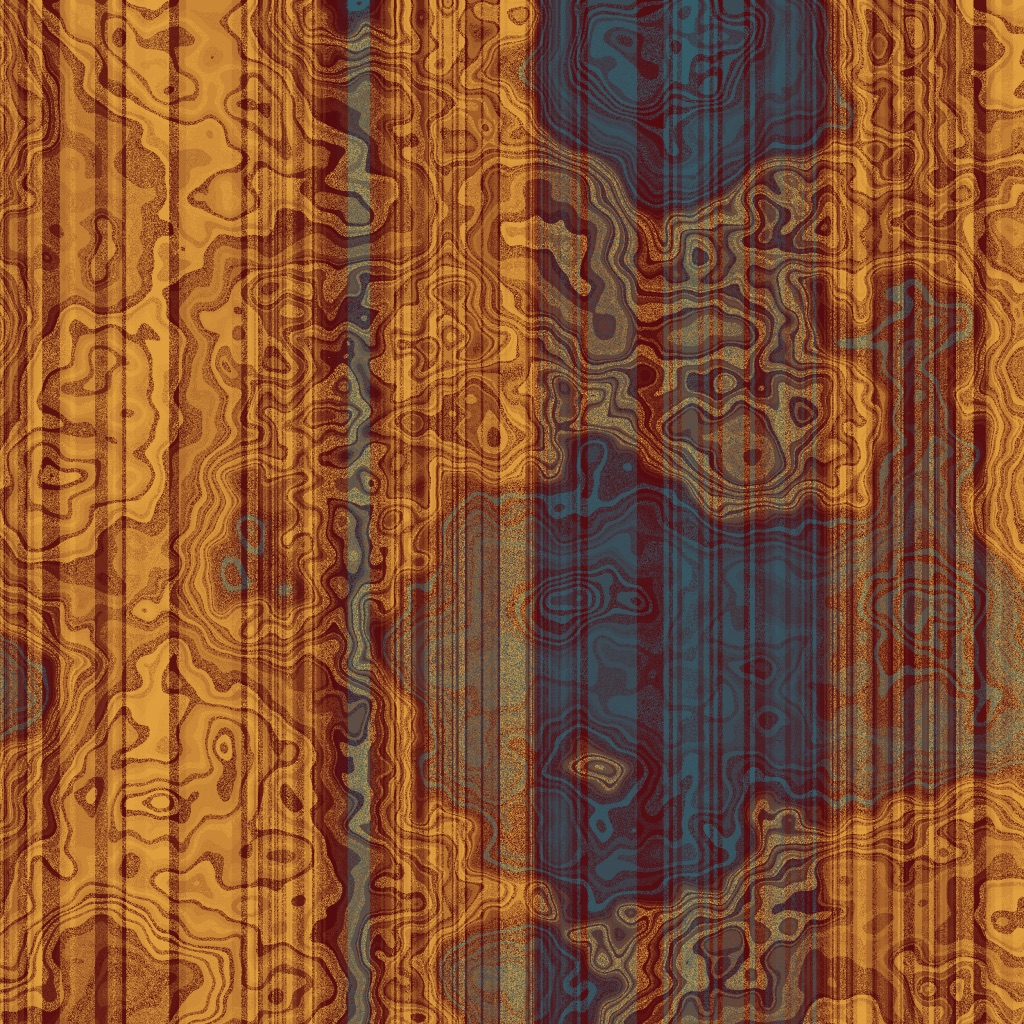
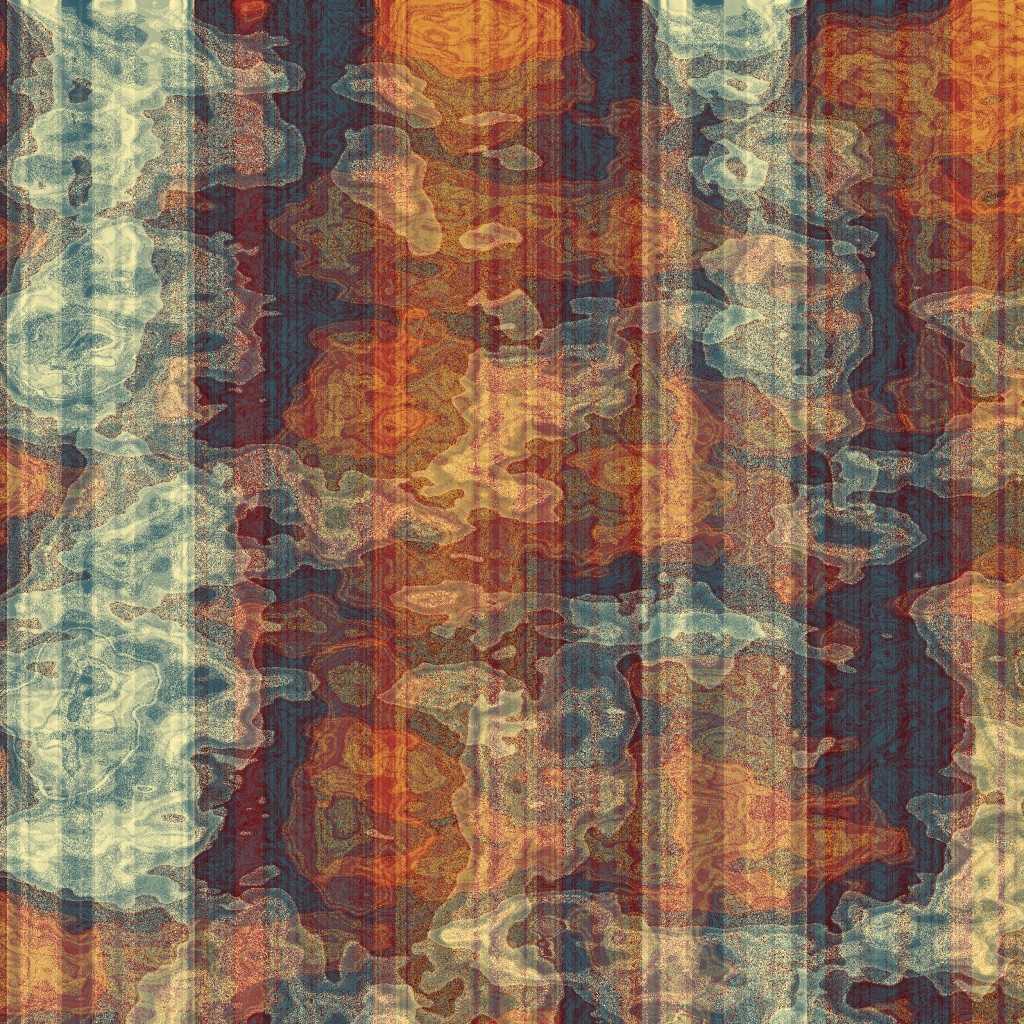
Full code can be found here:
https://github.com/jaminalder/staticart
starting point:
https://github.com/jaminalder/staticart/blob/master/src/staticart/sketch_8.clj